lightning:tree
component displays visualization of a structural hierarchy, such as a sitemap for a website or a role hierarchy in an organization. Items are displayed as hyperlinks and items in the hierarchy can be nested. Items with nested items are also known as branches.
To create a tree, we have to pass in an array of key-value pairs to the items attribute.
Below are the keys:
disabled
(Boolean): Specifies whether a branch is disabled. A disabled branch can’t be expanded. The default is false.
expanded
(Boolean): Specifies whether a branch is expanded. An expanded branch displays its nested items visually. The default is false.
href
(String): The URL of the link.
name
(String): The unique name for the item for the onselect
event handler to return the tree item that was clicked.
items
(Object): Nested items as an array of key-value pairs.
label
(String): Required. The title and label for the hyperlink.
Here is an example of Lightning Tree with list of accounts and respective contacts.
Apex Controller:
public class TreeAuraController {
@AuraEnabled
public static List<item> getAccountTree(){
List<item> items = new List<item>();
List<Account> acctList = new List<Account>();
//get list of accounts and respective contacts
acctList = [SELECT Id, Name, (SELECT Id, Name From Contacts) From Account LIMIT 10];
for(Account acc: acctList){
//get contacts of current account record
List<item> conitems = new List<item>();
for(Contact c: acc.Contacts){
//add contact items
item conitem = new item(c.Name, String.valueOf(c.Id), false, null);
conitems.add(conitem);
}
//add account items
item accitem = new item(acc.Name, String.valueOf(acc.Id), false, conitems);
items.add(accitem);
}
return items;
}
//Item Wrapper Class
public class item{
@AuraEnabled
public String label {get; set;}
@AuraEnabled
public String name {get; set;}
@AuraEnabled
public Boolean expanded {get; set;}
@AuraEnabled
public List<item> items {get; set;}
public item(String label, String name, Boolean expanded, List<item> items){
this.label = label;
this.name = name;
this.expanded = expanded;
this.items = items;
}
}
}
Lightning Component:
<!--Tree.cmp-->
<aura:component controller="TreeAuraController">
<aura:handler name="init" value="{!this}" action="{!c.doInit}"/>
<aura:attribute name="items" type="Object"/>
<!--Lightning Tree-->
<div class="slds-m-around_xx-large">
<lightning:tree items="{!v.items}" onselect="{!c.handleSelect}" header="Account and Contacts"/>
</div>
<!--Lightning Spinner-->
<div>
<lightning:spinner alternativeText="Processing.." title="Processing.." aura:id="spnr" variant="brand" size="large" />
</div>
</aura:component>
Lightning Component JS Controller:
({
doInit: function (component, event, helper) {
var spinner = component.find("spnr");
var action = component.get('c.getAccountTree');
action.setCallback(this, function(response){
var state = response.getState();
if(state === 'SUCCESS'){
//get account and respective contact list, and initialize with items
component.set('v.items', response.getReturnValue());
//hide spinner after getting data
$A.util.toggleClass(spinner, "slds-hide");
}else{
$A.util.toggleClass(spinner, "slds-hide");
alert('ERROR');
}
});
$A.enqueueAction(action);
},
handleSelect: function (cmp, event, helper) {
//return name of selected tree item
var selectedName = event.getParam('name');
alert("Selected Name: " + selectedName);
}
})
Lightning Test App:
<!--Test.app-->
<aura:application extends="force:slds">
<c:Tree />
</aura:application>
Output:
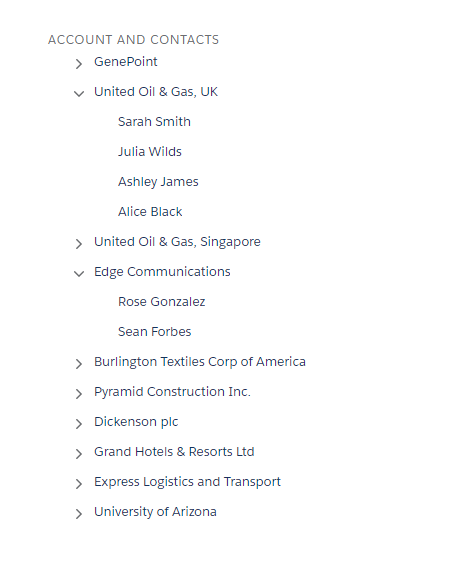